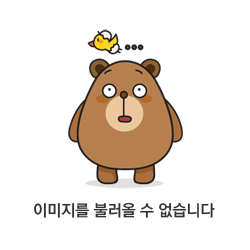
Spring 입문 주차 8.12(금) ~ 8.18(목)
1. 개인 과제: 게시판 CRUD 기능을 하는 백엔드 서버 만들기, AWS 배포 2. 팀 과제: Spring 핵심 키워드 정리(필수 4개, 선택 4개) |
진행 계획
- 12일(금) Spring 핵심 키워드 정리
- 13일(토) Spring 핵심 키워드 정리
- 14일(일) 개인과제에 필요한 강의 수강 및 공부
- 15일(월) API 명세서 작성, Use Case 그리기
- 16일(화) 게시판 CRUD 기능 코드 작성
- 17일(수) 게시판 CRUD 기능 코드 작성
- 18일(목) 게시판 CRUD 기능 코드 작성 및 AWS 배포
1. 개인 과제 진행 상황
1) 게시판 CRUD 기능 구현 완료 후 CommandLineRunner를 이용해서 테스트
@EnableJpaAuditing // JPA에서 DB가 변경되는 것을 감지하여 시간 자동 변경하도록 함
@SpringBootApplication // 스프링 부트임을 선언
public class Task03Application {
public static void main(String[] args) {
SpringApplication.run(Task03Application.class, args);
}
// 어플리케이션이 구동된 후 코드 실행
@Bean
public CommandLineRunner demo(PostRepository postRepository, PostService postService) {
return (args) -> {
postRepository.save(new Post(new PostRequestDto("제목", "내용", "작성자", "비밀번호"))); // 데이터 생성(저장)
// 게시글 전체 조회
System.out.println("데이터 인쇄");
List<Post> postList = postRepository.findAll();
for (int i=0; i<postList.size(); i++) {
Post post = postList.get(i);
System.out.println(post.getId());
System.out.println(post.getTitle());
System.out.println(post.getContent());
System.out.println(post.getUsername());
System.out.println(post.getPassword());
}
// 게시글 수정
PostRequestDto postRequestDto = new PostRequestDto("제목", "내용", "작성자", "비밀번호");
postService.updatePost(1L, postRequestDto);
postList = postRepository.findAll();
for (int i=0; i<postList.size(); i++) {
Post post = postList.get(i);
System.out.println(post.getId());
System.out.println(post.getTitle());
System.out.println(post.getContent());
System.out.println(post.getUsername());
System.out.println(post.getPassword());
}
// 게시글 삭제
postRepository.deleteAll();
};
}
}
2) AWS 배포
- RDS 연결: MySQL 이용
- EC2 배포: Ubuntu EC2 를 구매한 뒤, 8080 포트와 80번 포트를 연결하여 포트 번호 없이도 서비스에 접속 가능하게 하기
2. 개발 중 발생한 이슈와 해결
1) Web server failed to start. port 8080 was already in use에러
-> 포트가 이미 실행 중일 때 스프링을 Run하면 발생하는 에러
Web server failed to start. Port 3000 was already in use. Action:Identify and stop the process that's listening on port 3000 or configure this application to listen on another port.
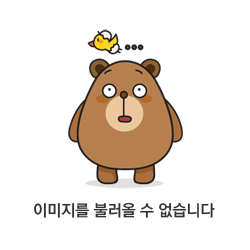
해결 방법: 터미널 -> lsof -i tcp:8080 -> sudo kill -9 xxxx(x자리에 PID번호 넣어주면 됨)
해당 프로세스를 강제 종료 시켜서 에러 해결
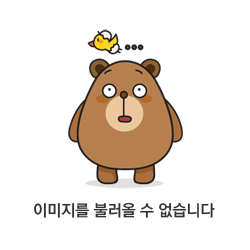
2) 포트 포워딩 에러(포트 80에서 8080으로 리다이렉트 설정할 때 발생한 에러)
web server failed to start. port 8080 was already in use.
-> 웹 서버 시작 실패. 포트 8080은 이미 사용됨
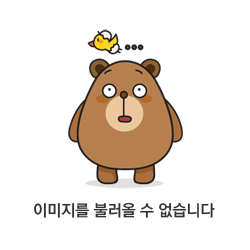
해결방법
원인은 전 프로젝트 때 포트 80에서 포트5000으로 리다이렉트 설정했던 것 때문
//현재 포워딩한 포트 확인
sudo iptables -t nat -L
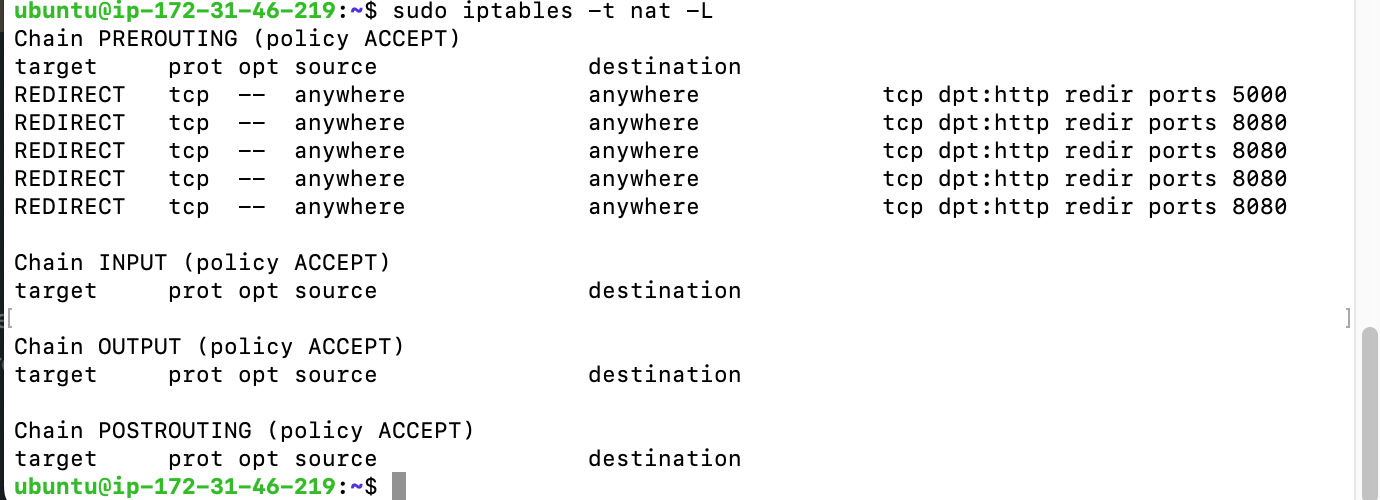
//포트80에서 포트 5000 리다이렉트 제거 명령
sudo iptables -D PREROUTING -t nat -i eth0 -p tcp --dport 80 -j REDIRECT --to-port 5000
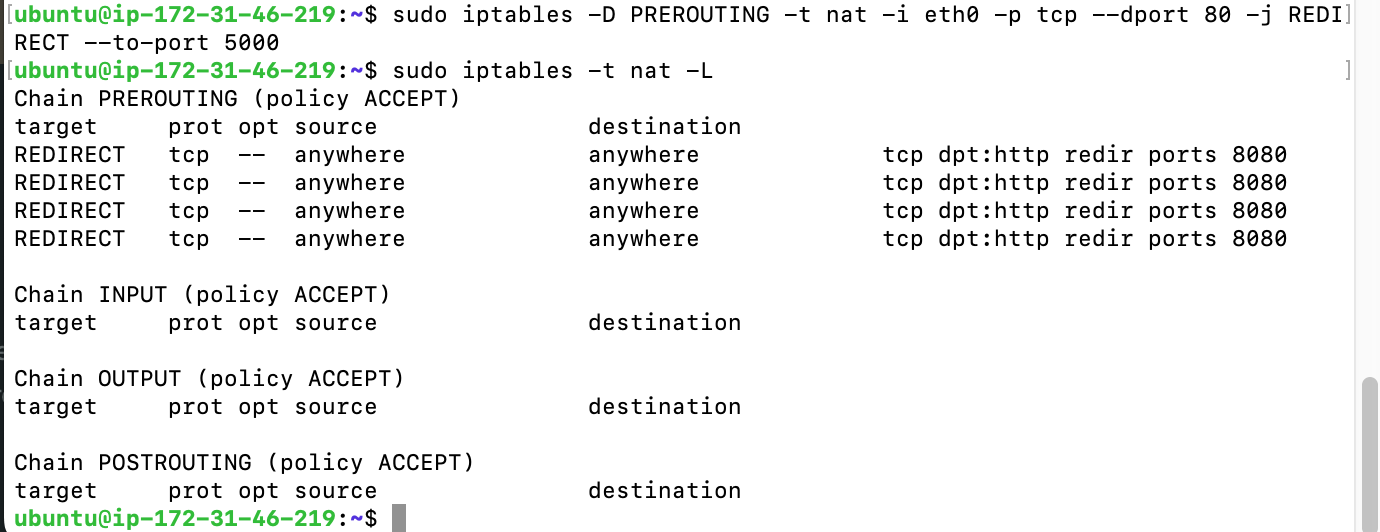
3) EC2(가상서버)에 올리는 중 jdk 버전 문제
파일질라에 jar파일 올리고 -> 터미널에서 스프링 부트 작동 시킴 -> 에러 발생
Application has been compiled by a more recent version of the Java Runtime(class file version 55.0), this version of the Java Runtime only recognizes class file version up to 52.0
-> (서버에 올릴려는 나의)어플리케이션 파일은 최신 버전(55.0=11version)으로 컴파일되었는데, 이 실행 버전은 52.0=8version클래스 파일까지만 인식
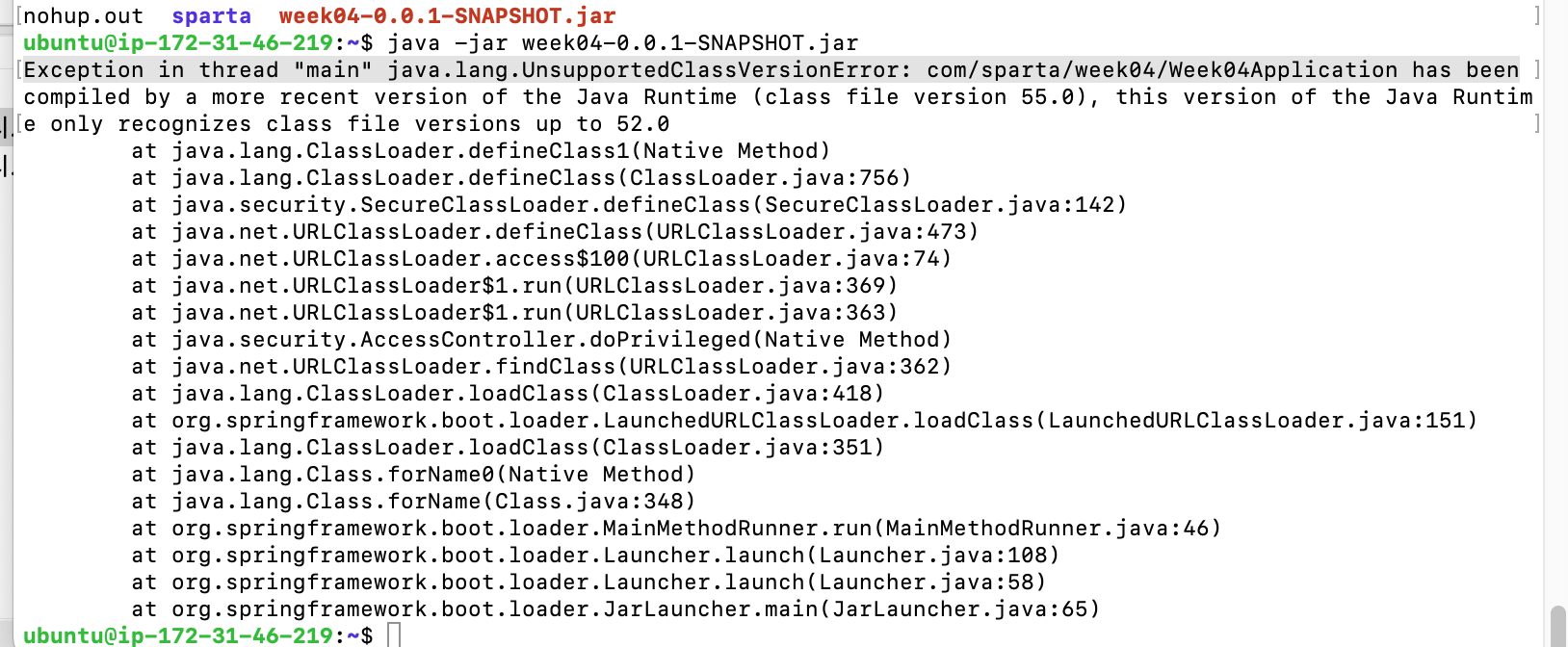
원인: 프로젝트 만들 때 설정했던 jdk 버전과 컴파일 버전이 맞지 않아서 발생한 에러
해결방법: jkd 11 설치

// jdk 11 버전 설치
sudo apt-get install openjdk-11-jdk

*** System restart required ***
Last login: Tue Aug 16 05:45:46 2022 from 125.179.147.69
ubuntu@ip-172-31-46-219:~$ sudo update
sudo: update: command not found
ubuntu@ip-172-31-46-219:~$ sudo apt-get install open-11-jdk
Reading package lists... Done
Building dependency tree... Done
Reading state information... Done
E: Unable to locate package open-11-jdk
ubuntu@ip-172-31-46-219:~$ java -version
openjdk version "11.0.16" 2022-07-19
OpenJDK Runtime Environment (build 11.0.16+8-post-Ubuntu-0ubuntu122.04)
OpenJDK 64-Bit Server VM (build 11.0.16+8-post-Ubuntu-0ubuntu122.04, mixed mode, sharing)
ubuntu@ip-172-31-46-219:~$ ls
nohup.out sparta week04-0.0.1-SNAPSHOT.jar
ubuntu@ip-172-31-46-219:~$ java -jar week04-0.0.1-SNAPSHOT.jar
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.7.2)
3. 오늘 한 일 / 회고
- 개인 과제: 테스트 코드 구현
- AWS 배포 완료
- Spring 입문 주차 과제 제출 완료
4. TO-DO LIST
- Spring 숙련 주차 과제
'개발 일지' 카테고리의 다른 글
[TIL]이노베이션 캠프 20일차 (0) | 2022.08.20 |
---|---|
[TIL]이노베이션 캠프 19일차 (0) | 2022.08.19 |
[TIL]이노베이션 캠프 17일차 (0) | 2022.08.17 |
[TIL]이노베이션 캠프 16일차 (0) | 2022.08.16 |
[TIL]이노베이션 캠프 15일차 (0) | 2022.08.15 |