1. HTTP 메시지 이해
Client와 Server 간 Request, Response는 HTTP 메시지 규약을 따름
2. HTTP Request와 Response 구성
1) Request
구조 | 내용 | 설명 | |
시작줄(start line) | GET naver.com HTTP/1.1 | API 호출, URL주소, HTTP/버전 | |
헤더(headers) | Content-Type | 없음 | - |
application/x-www-form-urlencoded | HTML | ||
application/json | AJAX | ||
본문(body) | (보통) 내용 없음 | GET요청 시 | |
(보통) 사용자가 입력한 폼 데이터 또는 json데이터 | POST요청 시 |
2) Response
구조 | 내용 | 설명 | |
상태줄( status line) | HTTP/1.1 404 Not Found | API 요청 결과(HTTP/버전, 상태 코드, 상태 텍스트) | |
헤더(headers) | Content-Type | 없음 | - |
text/html | HTML | ||
application/json | AJAX | ||
Location | http://localhost:8080/hello.html | Redirect할 페이지 URL | |
본문(body) | <!DOCTYPE html> <html> <head><title>By @ResponseBody</title></head> <body>Hello, Spring 정적 웹 페이지!!</body> </html> |
컨텐츠 타입이 HTML일 경우 | |
{ "name":"홍길동", "age": 20 } |
컨텐츠 타입이 JSON일 경우 |
3. Controller와 HTTP Response 메시지
Controller | HTTP Response | |||
@ResponseBody | Return형 | Return값 | 헤더(Header) | 바디(Body) |
X | String | "{View name}" | Content-Type:text/html | /templates/{View name}.html |
"redirect:/{URL}" | Location:{Host URL}/{URL} | 없음 | ||
O | String | "{Text}" | Content-Type:text/html | "{Text}" |
String 외 | Java객체 | Content-Type:application/json | Java객체 -> JOSN 변환 |
1) 정적 웹페이지
- static폴더-html파일
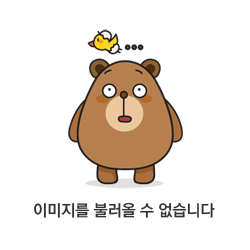
Url | 응답 |
http://localhost:8080/hello.html | ![]() |
- Redirect
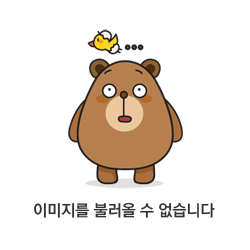
@Controller
@RequestMapping("/hello/response")
public class HelloResponseController {
@GetMapping("/html/redirect")
public String htmlFile() {
return "redirect:/hello.html";
}
}
Url | 응답 |
http://localhost:8080/hello/response/html/redirect -> redirect되어 http://localhost:8080/hello.html |
![]() 👉🏻 body 없음, html 응답 |
- Template engine인 타임리프에 View 전달
![]() |
![]() |
@GetMapping("/html/templates")
public String htmlTemplates() {
return "hello";
}
Url | 응답 |
http://localhost:8080/hello/response/html/templates -> resources**/templates/hello.html** |
![]() 👉🏻 body: View(hello.HTML)의 text내용 응답 |
👉🏻 타임리프 default 설정
- prefix: classpath:/templates/
- suffix: .html
- @ResponseBody
@GetMapping("/body/html")
@ResponseBody
public String helloStringHTML() {
return "<!DOCTYPE html>" +
"<html>" +
"<head><title>By @ResponseBody</title></head>" +
"<body> Hello, 정적 웹 페이지!!</body>" +
"</html>";
}
Url | 응답 |
http://localhost:8080/hello/response/html/templates | ![]() 👉🏻 body: "{text}" |
2) 동적 웹페이지
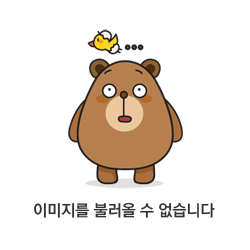
private static long visitCount = 0; //visitCount 초기값 0
@GetMapping("/html/dynamic")
public String helloHtmlFile(Model model) {
visitCount++; // visitCount 1씩 증가
model.addAttribute("visits", visitCount); // model 정보
return "hello-visit"; // view 정보
}
View, Model 정보를 템플릿 엔진인 타임리프에게 전달
타임 리프
View 정보: hello-visit" → resources/templates/hello-visit.html
<div>
(방문자 수: <span th:text="${visits}"></span>) // 타임리프 문법 th:
</div>
Model 정보: visits: 방문 횟수 (visitCount)
Url | 응답 |
http://localhost:8080/hello/response/html/dynamic | ![]() |
3) JSON데이터
@GetMapping("/json/string")
@ResponseBody
public String helloStringJson() {
return "{\"name\":\"BTS\",\"age\":28}";
}
요청 Url | 응답 헤더 | 응답 |
http://localhost:8080/hello/response/json/string | ![]() |
![]() |
👉🏻 JSON형식으로 응답받은 것 같아 보이지만 Content-Type이 text/html로 설정되어 있어 HTML이라고 인식하고 응답해준 나쁜 예
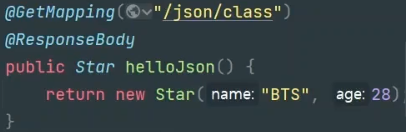
@GetMapping("/json/class")
@ResponseBody
public Star helloJson() {
return new Star("BTS", 28);
}
요청 Url | 응답 헤더 | 응답 |
http://localhost:8080/hello/response/json/class | ![]() |
![]() |
👉🏻 Content-Type가 application/json으로 설정되어 있어는 좋은 예
레퍼런스
https://spartacodingclub.kr/online/spring/plus
스파르타코딩클럽 [Spring 심화반]
Spring 뽀개고 취업하기
spartacodingclub.kr
'Spring > Springboot' 카테고리의 다른 글
Controller, Service, Repository의 역할 (0) | 2022.11.08 |
---|---|
Controller와 HTTP Request 메시지 (0) | 2022.11.08 |
빌드 관리 도구 Maven과 Gradle 비교 (0) | 2022.11.08 |
AOP(Aspect-Oriented Programming) 관점 지향 프로그래밍 구현 (1) | 2022.11.08 |
CORS(Cross-Origin Request) 알아보기 (0) | 2022.09.04 |